react-transition-state
Zero dependency React transition state machine
README
React-Transition-State
Features
Inspired by the React Transition Group, this tiny library helps you easily perform animations/transitions of your React component in a fully controlled manner, using a Hook API.
- 🍭 Working with both CSS animation and transition.
- 🔄 Moving React components in and out of DOM seamlessly.
- 🚫 Using no derived state.
- 🚀 Efficient: each state transition results in at most one extract render for consuming component.
- 🤏 Tiny: ~1KB(post-treeshaking) and no dependencies, ideal for both component libraries and applications.
🤔 Not convinced? See a comparison with _React Transition Group_
State diagram
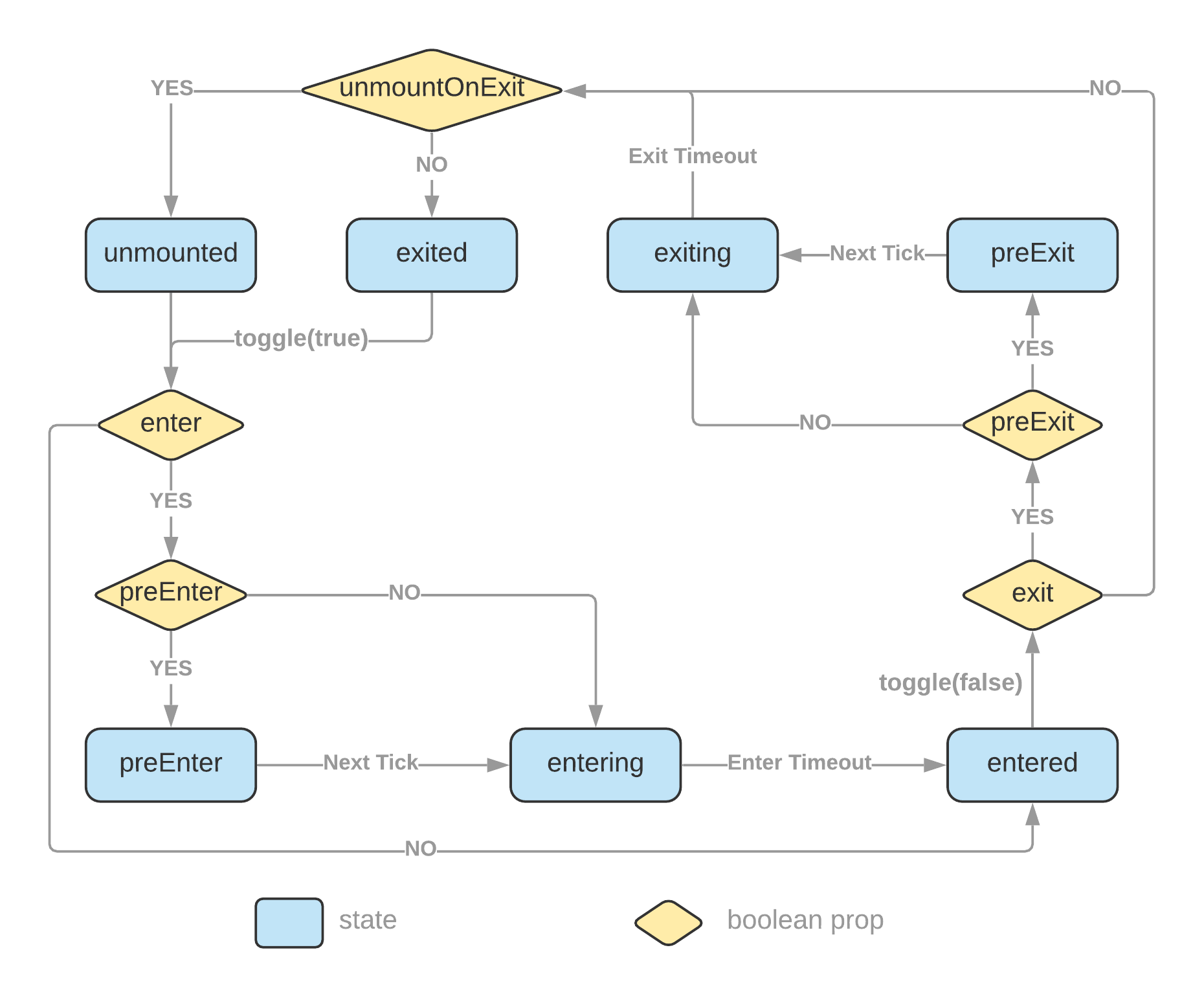
Install
- ```bash
- # with npm
- npm install react-transition-state
- # with Yarn
- yarn add react-transition-state
- ```
Usage
CSS example
- ```jsx
- import { useTransition } from 'react-transition-state';
- /* or import useTransition from 'react-transition-state'; */
- function Example() {
- const [state, toggle] = useTransition({ timeout: 750, preEnter: true });
- return (
- <div>
- <button onClick={() => toggle()}>toggle</button>
- <div className={`example ${state.status}`}>React transition state</div>
- </div>
- );
- }
- export default Example;
- ```
- ```css
- .example {
- transition: all 0.75s;
- }
- .example.preEnter,
- .example.exiting {
- opacity: 0;
- transform: scale(0.5);
- }
- .example.exited {
- display: none;
- }
- ```
styled-components example
- ```jsx
- import styled from 'styled-components';
- import { useTransition } from 'react-transition-state';
- const Box = styled.div`
- transition: all 500ms;
- ${({ status }) =>
- (status === 'preEnter' || status === 'exiting') &&
- `
- opacity: 0;
- transform: scale(0.9);
- `}
- `;
- function StyledExample() {
- const [{ status, isMounted }, toggle] = useTransition({
- timeout: 500,
- mountOnEnter: true,
- unmountOnExit: true,
- preEnter: true
- });
- return (
- <div>
- {!isMounted && <button onClick={() => toggle(true)}>Show Message</button>}
- {isMounted && (
- <Box status={status}>
- <p>This message is being transitioned in and out of the DOM.</p>
- <button onClick={() => toggle(false)}>Close</button>
- </Box>
- )}
- </div>
- );
- }
- export default StyledExample;
- ```
tailwindcss example
Perform appearing transition when page loads or a component mounts
You can toggle on transition with the useEffect hook.
- ```js
- useEffect(() => {
- toggle(true);
- }, [toggle]);
- ```
Comparisons with _React Transition Group_
| | This |
---|---|
--- | --- |
Use | _Yes_ |
Controlled | _No_ |
DOM | _Imperative_ |
Render | _Resort |
Working | Your |
Bundle | [](https://bundlephobia.com/package/react-transition-group) |
Dependency | [](https://www.npmjs.com/package/react-transition-group?activeTab=dependencies) |
This CodeSandbox example demonstrates how the same transition can be implemented in a simpler, more declarative, and controllable manner than _React Transition Group_.
API
useTransition Hook
- ```typescript
- function useTransition(
- options?: TransitionOptions
- ): [TransitionState, (toEnter?: boolean) => void, () => void];
- ```
Options
Name | Type | Default | Description |
---|---|---|---|
--- | --- | --- | --- |
`enter` | boolean | true | Enable |
`exit` | boolean | true | Enable |
`preEnter` | boolean | | | |
`preExit` | boolean | | | |
`initialEntered` | boolean | | | |
`mountOnEnter` | boolean | | | |
`unmountOnExit` | boolean | | | |
`timeout` | number | | | |
`onStateChange` | (event: | | |
Return value
The useTransition Hook returns a tuple of values in the following order:
1. state:
- ```js
- {
- status: 'preEnter' |
- 'entering' |
- 'entered' |
- 'preExit' |
- 'exiting' |
- 'exited' |
- 'unmounted';
- isMounted: boolean;
- isEnter: boolean;
- isResolved: boolean;
- }
- ```
2. toggle: (toEnter?: boolean) => void
- If no parameter is supplied, this function will toggle state between enter and exit phases.
- You can set a boolean parameter to explicitly switch into one of the two phases.
3. endTransition: () => void
- Call this function to stop transition which will turn state into 'entered' or 'exited'.
- You will normally call this function in the onAnimationEnd or onTransitionEnd event.
- You need to either call this function explicitly in your code or set a timeout value in Hook options.
useTransitionMap Hook
It's similar to the useTransition Hook except that it manages multiple states in a Map structure instead of a single state.
Options
It accepts all options as useTransition and the following ones:
Name | Type | Default | Description |
---|---|---|---|
--- | --- | --- | --- |
`allowMultiple` | boolean | | |
Return value
The Hook returns an object of shape:
- ```js
- interface TransitionMapResult<K> {
- stateMap: ReadonlyMap<K, TransitionState>;
- toggle: (key: K, toEnter?: boolean) => void;
- toggleAll: (toEnter?: boolean) => void;
- endTransition: (key: K) => void;
- setItem: (key: K, options?: TransitionItemOptions) => void;
- deleteItem: (key: K) => boolean;
- }
- ```
setItem and deleteItem are used to add and remove items from the state map.
License
MIT Licensed.