React Image Crop
A responsive image cropping tool for React
README
React Image Crop
An image cropping tool for React with no dependencies.
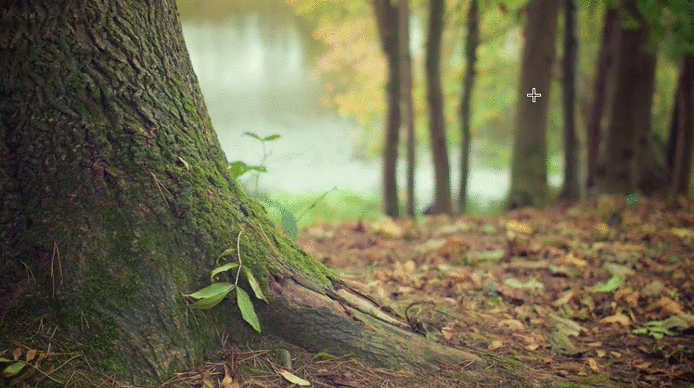
Features
- Responsive (you can use pixels or percentages).
- Touch enabled.
- Free-form or fixed aspect crops.
- Fully keyboard accessible (a11y).
- No dependencies/small footprint (<5KB gzip).
- Min/max crop size.
- Crop anything, not just images.
Installation
- ```
- npm i react-image-crop --save
- yarn add react-image-crop
- pnpm add react-image-crop
- ```
This library works with all modern browsers. It does not work with IE.
Usage
Include the main js module:
- ```js
- import ReactCrop from 'react-image-crop'
- ```
Include either dist/ReactCrop.css or ReactCrop.scss.
- ```js
- import 'react-image-crop/dist/ReactCrop.css'
- // or scss:
- import 'react-image-crop/src/ReactCrop.scss'
- ```
Example
- ```tsx
- import ReactCrop, { type Crop } from 'react-image-crop'
- function CropDemo({ src }) {
- const [crop, setCrop] = useState<Crop>()
- return (
- <ReactCrop crop={crop} onChange={c => setCrop(c)}>
- <img src={src} />
- </ReactCrop>
- )
- }
- ```
See the sandbox demo for a more complete example.
CDN
- ```html
- <link href="https://unpkg.com/react-image-crop/dist/ReactCrop.css" rel="stylesheet" />
- <script src="https://unpkg.com/react-image-crop/dist/index.umd.cjs"></script>
- ```