PlayCanvas
Fast and lightweight JavaScript game engine built on WebGL and glTF
README
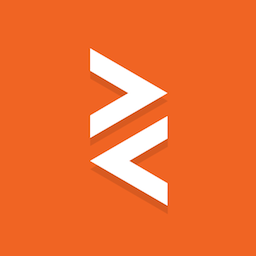
PlayCanvas WebGL Game Engine
PlayCanvas is an open-source game engine. It uses HTML5 and WebGL to run games and other interactive 3D content in any mobile or desktop browser.
[![NPM version][npm-badge]][npm-url] [![Minzipped size][minzip-badge]][minzip-url] [![Language grade: JavaScript][code-quality-badge]][code-quality-url] [![Average time to resolve an issue][resolution-badge]][isitmaintained-url] [![Percentage of issues still open][open-issues-badge]][isitmaintained-url] [![Twitter][twitter-badge]][twitter-url]
Project Showcase
[Many games and apps](https://github.com/playcanvas/awesome-playcanvas#awesome-playcanvas-
) have been published using the PlayCanvas engine. Here is a small selection:
You can see more games on the PlayCanvas website.
Users
PlayCanvas is used by leading companies in video games, advertising and visualization such as:
Animech, Arm, BMW, Disney, Facebook, Famobi, Funday Factory, IGT, King, Miniclip, Leapfrog, Mojiworks, Mozilla, Nickelodeon, Nordeus, NOWWA, PikPok, PlaySide Studios, Polaris, Product Madness, Samsung, Snap, Spry Fox, Zeptolab, Zynga
Features
PlayCanvas is a fully featured game engine.
🧊 Graphics - Advanced 2D + 3D graphics engine built on WebGL 1 & 2.
🏃 Animation - Powerful state-based animations for characters and arbitrary scene properties
⚛️ Physics - Full integration with 3D rigid-body physics engine ammo.js
🎮 Input - Mouse, keyboard, touch, gamepad and VR controller APIs
🔊 Sound - 3D positional sounds built on the Web Audio API
📜 Scripts - Write game behaviors in Typescript or JavaScript
Usage
Here's a super-simple Hello World example - a spinning cube!
- ```html
- <!DOCTYPE html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>PlayCanvas Hello Cube</title>
- <meta name='viewport' content='width=device-width, initial-scale=1, maximum-scale=1, minimum-scale=1, user-scalable=no' />
- <style>
- body {
- margin: 0;
- overflow: hidden;
- }
- </style>
- <script src='https://code.playcanvas.com/playcanvas-stable.min.js'></script>
- </head>
- <body>
- <canvas id='application'></canvas>
- <script>
- // create a PlayCanvas application
- const canvas = document.getElementById('application');
- const app = new pc.Application(canvas);
- // fill the available space at full resolution
- app.setCanvasFillMode(pc.FILLMODE_FILL_WINDOW);
- app.setCanvasResolution(pc.RESOLUTION_AUTO);
- // ensure canvas is resized when window changes size
- window.addEventListener('resize', () => app.resizeCanvas());
- // create box entity
- const box = new pc.Entity('cube');
- box.addComponent('model', {
- type: 'box'
- });
- app.root.addChild(box);
- // create camera entity
- const camera = new pc.Entity('camera');
- camera.addComponent('camera', {
- clearColor: new pc.Color(0.1, 0.1, 0.1)
- });
- app.root.addChild(camera);
- camera.setPosition(0, 0, 3);
- // create directional light entity
- const light = new pc.Entity('light');
- light.addComponent('light');
- app.root.addChild(light);
- light.setEulerAngles(45, 0, 0);
- // rotate the box according to the delta time since the last frame
- app.on('update', dt => box.rotate(10 * dt, 20 * dt, 30 * dt));
- app.start();
- </script>
- </body>
- </html>
- ```
Want to play with the code yourself? Edit it on CodePen.
How to build
Ensure you have Node.js installed. Then, install all of the required Node.js dependencies:
npm install
Now you can run various build options:
Command | Description | Outputs |
---|---|---|
|-----------------------|-------------------------------------------|----------------------------------| | ||
`npm | Build | `build\playcanvas[.min/.dbg/.prf].[mjs/js]` |
`npm | Build | `build\playcanvas[.min/.dbg/.prf].js` |
`npm | Build | `build\playcanvas.[mjs/js]` |
`npm | Build | `build\playcanvas.d.ts` |
`npm | Build | `docs` |
Pre-built versions of the engine are also available.
Latest development release (head revision of dev branch):
https://code.playcanvas.com/playcanvas-latest.js
https://code.playcanvas.com/playcanvas-latest.min.js
Latest stable release:
https://code.playcanvas.com/playcanvas-stable.js
https://code.playcanvas.com/playcanvas-stable.min.js
Specific engine versions:
https://code.playcanvas.com/playcanvas-1.38.4.js
https://code.playcanvas.com/playcanvas-1.38.4.min.js
Generate Source Maps
To build the source map to allow for easier engine debugging, you can add -- -m to any engine build command. For example:
npm run build -- -m
This will output to build/playcanvas.js.map
PlayCanvas Editor
The PlayCanvas Engine is an open source engine which you can use to create HTML5 apps/games. In addition to the engine, we also make the PlayCanvas Editor:
For Editor related bugs and issues, please refer to the Editor's repo.
[npm-badge]: https://img.shields.io/npm/v/playcanvas
[npm-url]: https://www.npmjs.com/package/playcanvas
[minzip-badge]: https://img.shields.io/bundlephobia/minzip/playcanvas
[minzip-url]: https://bundlephobia.com/result?p=playcanvas
[code-quality-badge]: https://img.shields.io/lgtm/grade/javascript/g/playcanvas/engine.svg?logo=lgtm&logoWidth=18
[code-quality-url]: https://lgtm.com/projects/g/playcanvas/engine/context:javascript
[resolution-badge]: https://isitmaintained.com/badge/resolution/playcanvas/engine.svg
[open-issues-badge]: https://isitmaintained.com/badge/open/playcanvas/engine.svg
[isitmaintained-url]: https://isitmaintained.com/project/playcanvas/engine
[twitter-badge]: https://img.shields.io/twitter/follow/playcanvas.svg?style=social&label=Follow
[twitter-url]: https://twitter.com/intent/follow?screen_name=playcanvas
[docs]: https://developer.playcanvas.com/en/api/