react-archer
Draw arrows between React elements
README
react-archer
🏹 Draw arrows between DOM elements in React 🖋
Installation
npm install react-archer --save or yarn add react-archer
Example
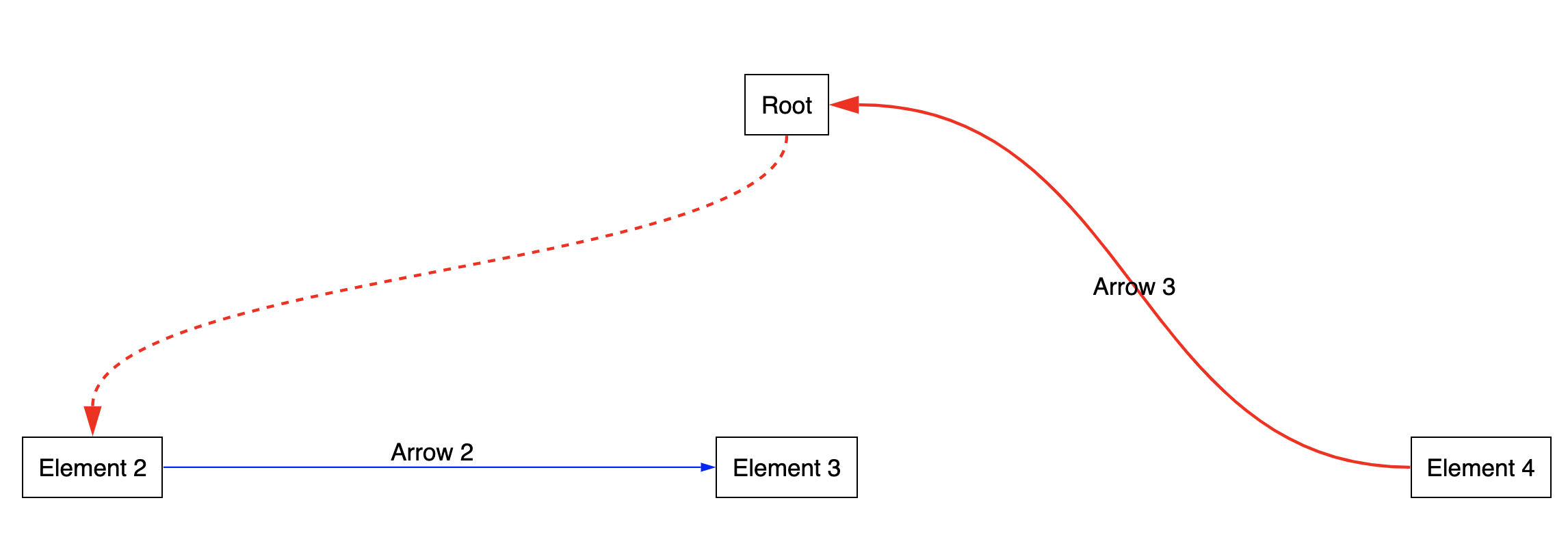
- ```javascript
- import { ArcherContainer, ArcherElement } from 'react-archer';
- const rootStyle = { display: 'flex', justifyContent: 'center' };
- const rowStyle = { margin: '200px 0', display: 'flex', justifyContent: 'space-between' };
- const boxStyle = { padding: '10px', border: '1px solid black' };
- const App = () => {
- return (
- <div style={{ height: '500px', margin: '50px' }}>
- <ArcherContainer strokeColor="red">
- <div style={rootStyle}>
- <ArcherElement
- id="root"
- relations={[
- {
- targetId: 'element2',
- targetAnchor: 'top',
- sourceAnchor: 'bottom',
- style: { strokeDasharray: '5,5' },
- },
- ]}
- >
- <div style={boxStyle}>Root</div>
- </ArcherElement>
- </div>
- <div style={rowStyle}>
- <ArcherElement
- id="element2"
- relations={[
- {
- targetId: 'element3',
- targetAnchor: 'left',
- sourceAnchor: 'right',
- style: { strokeColor: 'blue', strokeWidth: 1 },
- label: <div style={{ marginTop: '-20px' }}>Arrow 2</div>,
- },
- ]}
- >
- <div style={boxStyle}>Element 2</div>
- </ArcherElement>
- <ArcherElement id="element3">
- <div style={boxStyle}>Element 3</div>
- </ArcherElement>
- <ArcherElement
- id="element4"
- relations={[
- {
- targetId: 'root',
- targetAnchor: 'right',
- sourceAnchor: 'left',
- label: 'Arrow 3',
- },
- ]}
- >
- <div style={boxStyle}>Element 4</div>
- </ArcherElement>
- </div>
- </ArcherContainer>
- </div>
- );
- };
- export default App;
- ```
API
ArcherContainer
Props
Name | Type | Description |
---|---|---|
- | - | - |
`strokeColor` | `string` | A |
`strokeWidth` | `number` | A |
`strokeDasharray` | `string` | Adds |
`noCurves` | `boolean` | Set |
`lineStyle` | `string` | Can |
`offset` | `number` | Optional |
`svgContainerStyle` | `Style` | Style |
`children` | `React.Node` | |
`endShape` | `Object` | An |
`startMarker` | `boolean` | Optional |
`endMarker` | `boolean` | Optional |
Instance methods
If you access to the ref of your ArcherContainer, you will access the refreshScreen method.
This will allow you to have more control on when you want to re-draw the arrows.
Troubleshooting
My arrows don't re-render correctly...
Try using the refreshScreen instance method on your ArcherContainer element. You can access it through the ref of the component.
Call refreshScreen when the event that you need is triggered (onScroll etc.).