Flitter
A powerful framework inspired by Flutter, supporting both SVG and Canvas to...
README
Flitter
Flitter is a powerful framework inspired by Flutter, supporting both SVG and Canvas to create high-performance graphics and user interfaces. It is designed to easily implement complex data visualizations, interactive charts, diagrams, and graphic editors in web applications.
Key Features
- Render Object Tree: Flitter uses a render object tree for efficient rendering, allowing easy management and manipulation of complex layouts.
- Declarative Programming: Following a declarative paradigm, the screen automatically updates when values change, simplifying application state management.
- Optimized Rendering: Re-rendering, painting, and layout recalculations are managed by the renderer pipeline, with optimizations applied to update only necessary parts.
- Box Model Layout: Users can easily compose layouts using the familiar Box model.
- SVG and Canvas Support: Supports both SVG and Canvas, meeting various graphic requirements. Developers can choose the appropriate renderer as needed.
- Diverse Applications: Can be utilized in various fields such as charts, diagrams, data visualization, and graphic editors.
Showcase
Here are some examples of what you can create with Flitter:
Interactive ERD (Entity-Relationship Diagram)[https://easyrd.dev]
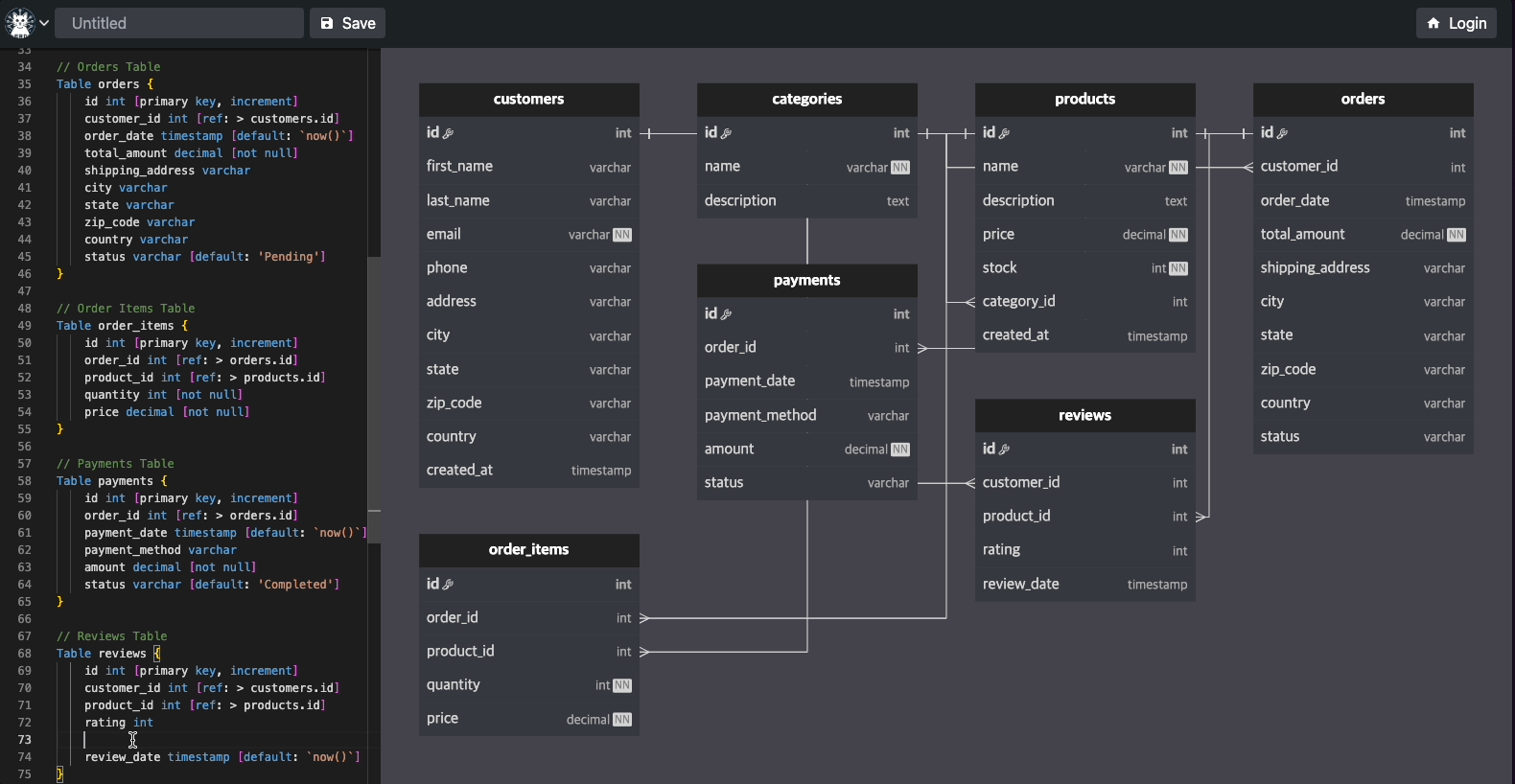
This interactive ERD demonstrates Flitter's capability to create complex, interactive diagrams. Users can manipulate entities, add relationships, and visualize database structures in real-time. This showcase highlights Flitter's strengths in:
Creating responsive, draggable elements
Handling complex user interactions
Rendering intricate diagrams with ease
Real-time updates based on user input
Installation Guide
Flitter can be used in various JavaScript environments. Here are installation and usage methods for major environments:
Pure JavaScript
- ```bash
- npm install @meursyphus/flitter
- ```
- ```javascript
- import { Widget, Container, AppRunner } from '@meursyphus/flitter';
- // Using SVG renderer
- const svgElement = document.getElementById('mySvg');
- const svgRunner = new AppRunner({ view: svgElement });
- svgRunner.runApp(Container({ color: 'lightblue' }));
- // Using Canvas renderer
- const canvasElement = document.getElementById('myCanvas');
- const canvasRunner = new AppRunner({ view: canvasElement });
- canvasRunner.runApp(Container({ color: 'lightgreen' }));
- ```
React
- ```bash
- npm install @meursyphus/flitter @meursyphus/flitter-react
- ```
- ```jsx
- import { Container, Alignment, Text, TextStyle } from '@meursyphus/flitter';
- import Widget from '@meursyphus/flitter-react';
- const App = () => (
- <>
- <Widget
- width="600px"
- height="300px"
- renderer="svg"
- widget={Container({
- alignment: Alignment.center,
- color: 'lightblue',
- child: Text("Hello, Flitter SVG!", { style: TextStyle({ fontSize: 30, weight: 'bold' }) })
- })}
- />
- <Widget
- width="600px"
- height="300px"
- renderer="canvas"
- widget={Container({
- alignment: Alignment.center,
- color: 'lightgreen',
- child: Text("Hello, Flitter Canvas!", { style: TextStyle({ fontSize: 30, weight: 'bold' }) })
- })}
- />
- </>
- );
- ```