tslog
Beautiful logging experience for TypeScript and JavaScript
README
📝 tslog: Beautiful logging experience for TypeScript and JavaScript
Powerful, fast and expressive logging for TypeScript and JavaScript
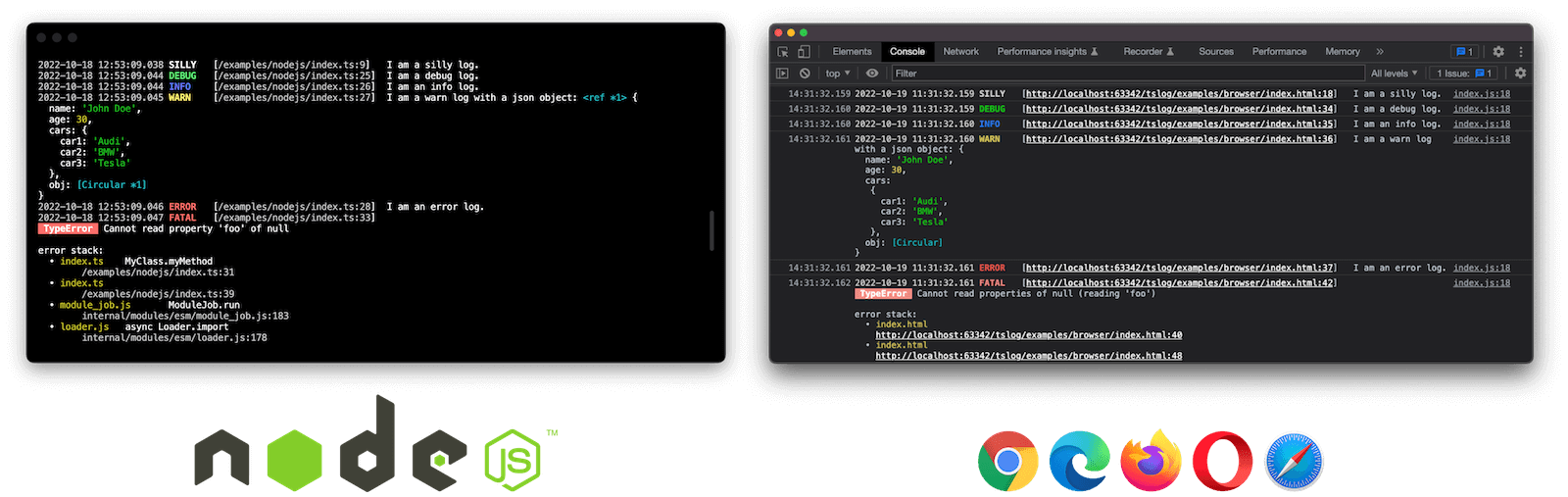
Highlights
🪶 **Lightweight and flexible**
🏗 **Universal: Works in Browsers and Node.js**
👮️ **Fully typed with TypeScript support (native source maps)**
🗃 **_Pretty_ or `JSON` output**
📝 **Customizable log level**
⭕️ **Supports _circular_ structures**
🦸 **Custom pluggable loggers**
💅 **Object and error interpolation**
🤓 **Stack trace and pretty errors**
👨👧👦 **Sub-logger with inheritance**
🙊 **Mask/hide secrets and keys**
📦 **CJS & ESM with tree shaking support**
✍️ **Well documented and tested**
Example
- ```typescript
- import { Logger } from "tslog";
- const log: Logger = new Logger();
- log.silly("I am a silly log.");
- ```
Donations help me allocate more time for my open source work.
Install
- ``` sh
- npm install tslog
- ```
In order to run a native ES module in Node.js, you have to do two things:
1) Set "type": "module" in package.json.
2) For now, start with --experimental-specifier-resolution=node
Example package.json
- ``` json5
- {
- "name": "NAME",
- "version": "1.0.0",
- "main": "index.js",
- // here:
- "type": "module",
- "scripts": {
- "build": "tsc -p .",
- // and here:
- "start": "node --enable-source-maps --experimental-specifier-resolution=node index.js"
- },
- "dependencies": {
- "tslog": "^4"
- },
- "devDependencies": {
- "typescript": "^4"
- },
- "engines": {
- "node": ">=16"
- }
- }
- ```
With this package.json you can simply build and run it:
- ``` sh
- npm run build
- npm start
- ```
Otherwise:
ESM: Node.js with JavaScript:
- ``` sh
- node --enable-source-maps --experimental-specifier-resolution=node
- ```
CJS: Node.js with JavaScript:
- ``` sh
- node --enable-source-maps
- ```
ESM: Node.js with TypeScript and ts-node:
- ``` sh
- node --enable-source-maps --experimental-specifier-resolution=node --no-warnings --loader ts-node/esm
- ```
CJS: Node.js with TypeScript and ts-node:
- ``` sh
- node --enable-source-maps --no-warnings --loader ts-node/cjs
- ```
Browser:
- ``` html
- <!doctype html>
- <html lang="en">
- <head>
- <title>tslog example</title>
- </head>
- <body>
- <h1>Example</h1>
- <script src="tslog.js"></script>
- <script>
- const logger = new tslog.Logger();
- logger.silly("I am a silly log.");
- </script>
- </body>
- </html>
- ```
Enable TypeScript source map support:
This feature enables tslog to reference a correct line number in your TypeScript source code.
- ``` json5
- // tsconfig.json
- {
- // ...
- compilerOptions: {
- // ...
- "inlineSourceMap": true, //