cote
A Node.js library for building zero-configuration microservices.
README
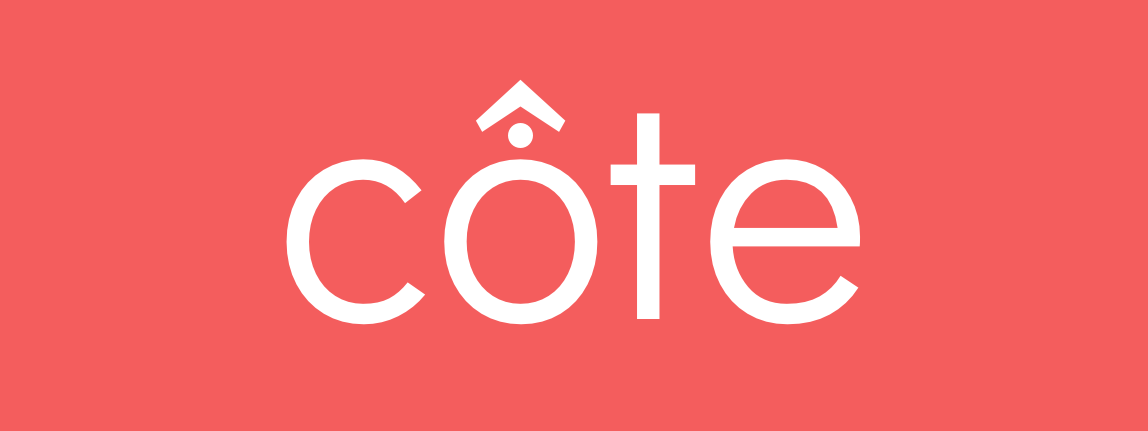
cote — A Node.js library for building zero-configuration microservices
cote lets you write zero-configuration microservices in Node.js without nginx,
haproxy, redis, rabbitmq or _anything else_. It is batteries — and chargers! —
included.
Join us on
for anything related to cote.
Features
- Zero dependency: Microservices with only JavaScript and Node.js
- Zero-configuration: No IP addresses, no ports, no routing to configure
- Decentralized: No fixed parts, no "manager" nodes, no single point of
failure
- Auto-discovery: Services discover each other without a central bookkeeper
- Fault-tolerant: Don't lose any requests when a service is down
- Scalable: Horizontally scale to any number of machines
- Performant: Process thousands of messages per second
- Humanized API: Extremely simple to get started with a reasonable API!
Develop your first microservices in under two minutes:
in time-service.js...
- ```js
- const cote = require('cote');
- const timeService = new cote.Responder({ name: 'Time Service' });
- timeService.on('time', (req, cb) => {
- cb(new Date());
- });
- ```
in client.js...
- ```js
- const cote = require('cote');
- const client = new cote.Requester({ name: 'Client' });
- client.send({ type: 'time' }, (time) => {
- console.log(time);
- });
- ```
You can run these files anyway you like — on a single machine or scaled out to
hundreds of machines in different datacenters — and they will just work. No
configuration, no third party components, no nginx, no kafka, no consul and
only Node.js. cote is batteries — and chargers — included!
Microservices case study
Make sure to check out
implements a complete e-commerce application with microservices using
cote. It features;
+ a back-office with real-time updates for managing the catalogue of products
and displaying sales with a RESTful API (express.js)
+ a storefront for end-users with real-time updates to products where they
can buy the products with WebSockets (socket.io)
+ a user microservice for user CRUD
+ a product microservice for product CRUD
+ a purchase microservice that enables users to buy products
+ a payment microservice that deals with money transactions that occur as
a result of purchases
+ Docker compose configuration for running the system locally
cote plays very well with Docker, taking advantage of its network overlay
features. The case study implements a scalable microservices application
via Docker and can scale to multiple machines.