Inputmask
Input Mask plugin
README
Inputmask
The Inputmask has a very permissive license and this will stay that way. But when you use the Inputmask in a commercial setting, be so honest to make a small donation.
This will be appreciated very much.
Inputmask is a javascript library that creates an input mask. Inputmask can run against vanilla javascript, jQuery, and jqlite.
An inputmask helps the user with the input by ensuring a predefined format. This can be useful for dates, numerics, phone numbers, ...
Highlights:
- easy to use
- optional parts anywhere in the mask
- possibility to define aliases which hide the complexity
- date / DateTime masks
- numeric masks
- lots of callbacks
- non-greedy masks
- many features can be enabled/disabled/configured by options
- supports read-only/disabled/dir="rtl" attributes
- support data-inputmask attribute(s)
- alternator-mask
- regex-mask
- dynamic-mask
- preprocessing-mask
- JIT-masking
- value formatting / validating without input element
- AMD/CommonJS support
- dependencyLibs: vanilla javascript, jQuery, jqlite
- \Thanks to Jetbrains for providing a free license for their excellent Webstorm IDE.
Thanks to Browserstack for providing a free license, so we can automate testing in different browsers and devices.
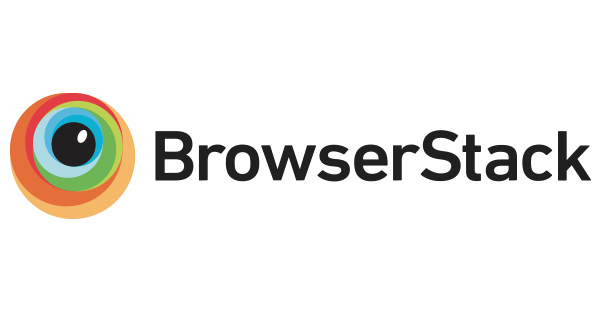
Setup
dependencyLibs
Inputmask can run against different javascript libraries.
You can choose between:
- inputmask.dependencyLib (vanilla)
- inputmask.dependencyLib.jquery