OverlayScrollbars
A javascript scrollbar plugin that hides native scrollbars, provides custom...
README
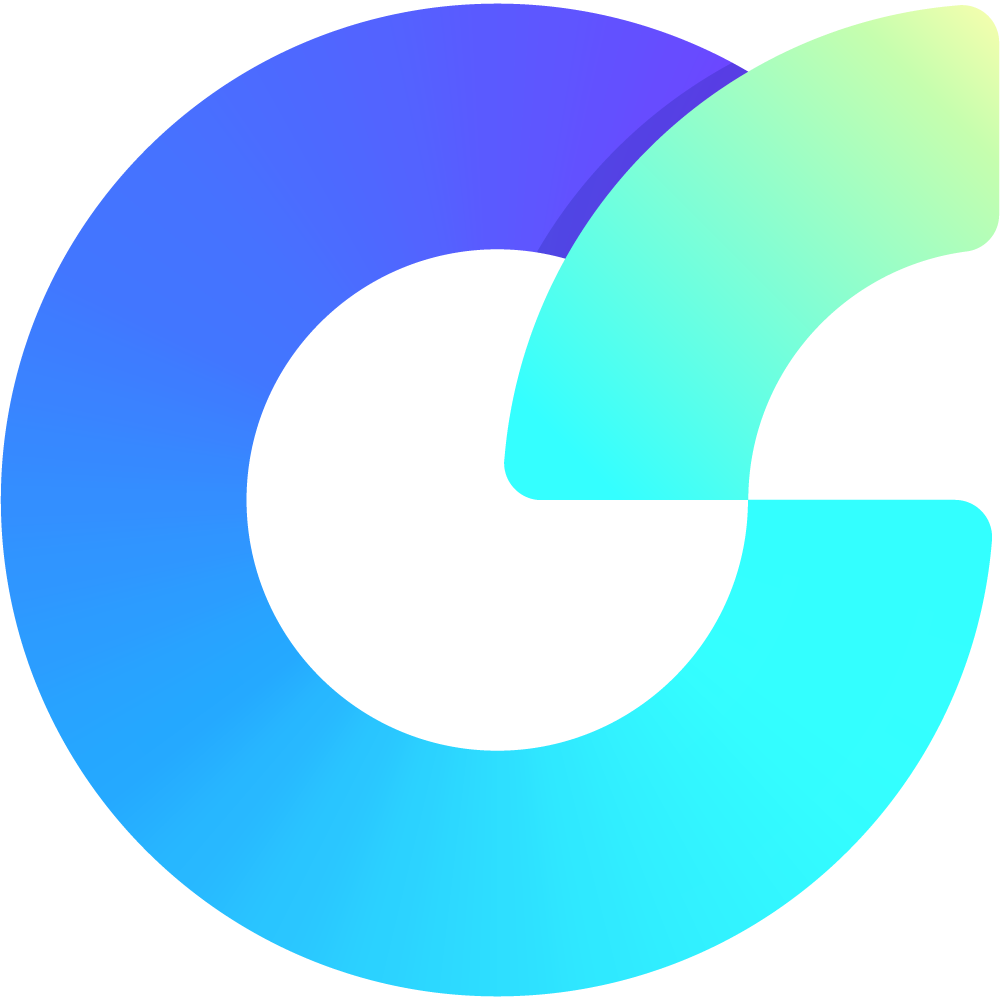
OverlayScrollbars
OverlayScrollbars is a javascript scrollbar plugin that hides native scrollbars, provides custom styleable overlay scrollbars and keeps the native functionality and feeling.
Why
I created this plugin because I hate ugly and space consuming scrollbars. Similar plugins haven't met my requirements in terms of features, quality, simplicity, license or browser support.
Goals & Features
- Simple, powerful and well documented API
- High browser compatibility - Firefox 59+, Chrome 55+, Opera 42+, Edge 15+ and Safari 10+
- Fully Accessible - Native scroll behavior is completely preserved
- Can be run on the server (Node, Deno and Bun) - SSR, SSG and ISR support
- Tested on various devices - Mobile, Desktop and Tablet
- Tested with various (and mixed) inputs - Mouse, Touch and Pen
- Treeshaking - bundle only what you really need
- Automatic update detection - no polling
- Usage of latest browser features - best performance in new browsers
- Flow independent - supports all values for direction, flex-direction and writing-mode
- Supports Scroll Snapping
- Supports all virtual scrolling libraries
- Supports the body element
- Simple and effective scrollbar styling
- Highly customizable
- TypeScript support - fully written in TypeScript
- Dependency free - 100% self written to ensure small size and best functionality
Getting started
npm & nodejs
OverlayScrollbars can be downloaded from npm or the package manager of your choice:
- ```sh
- npm install overlayscrollbars
- ```
After installation it can be imported:
- ```js
- import 'overlayscrollbars/overlayscrollbars.css';
- import {
- OverlayScrollbars,
- ScrollbarsHidingPlugin,
- SizeObserverPlugin,
- ClickScrollPlugin
- } from 'overlayscrollbars';
- ```
__Note__: If the path 'overlayscrollbars/overlayscrollbars.css' is not working use 'overlayscrollbars/styles/overlayscrollbars.css' as the import path for the CSS file.
You can use this Node Example as an reference / starting point.
Manual download & embedding
You can use OverlayScrollbars without any bundler or package manager.
- Use the javascript files with the .browser extension.
- Use the javascript files with the .es5 extension if you need to support older browsers, otherwise use the .es6 files.
- For production use the javascript / stylesheet files with the .min extension.
Embedd OverlayScrollbars manually in your HTML:
- ```html
- <link type="text/css" href="path/to/overlayscrollbars.css" rel="stylesheet" />
- <script type="text/javascript" src="path/to/overlayscrollbars.browser.es.js" defer></script>
- ```
Use the global variable OverlayScrollbarsGlobal to access the api similar to how you can do it in nodejs / modules:
- ```js
- var {
- OverlayScrollbars,
- ScrollbarsHidingPlugin,
- SizeObserverPlugin,
- ClickScrollPlugin
- } = OverlayScrollbarsGlobal;
- ```
You can use this Browser Example as an reference / starting point.
The examples in this documentation will use the import syntax instead of the OverlayScrollbarsGlobal object. Both versions are equivalent though.
Initialization
You can initialize either directly with an Element or with an Object where you have more control over the initialization process.
- ```js
- // simple initialization with an element
- const osInstance = OverlayScrollbars(document.querySelector('#myElement'), {});
- ```