Lozad
Highly performant, light ~1kb and configurable lazy loader in pure JS with ...
README
Highly performant, light and configurable lazy loader in pure JS with no dependencies for images, iframes and more, using IntersectionObserver API
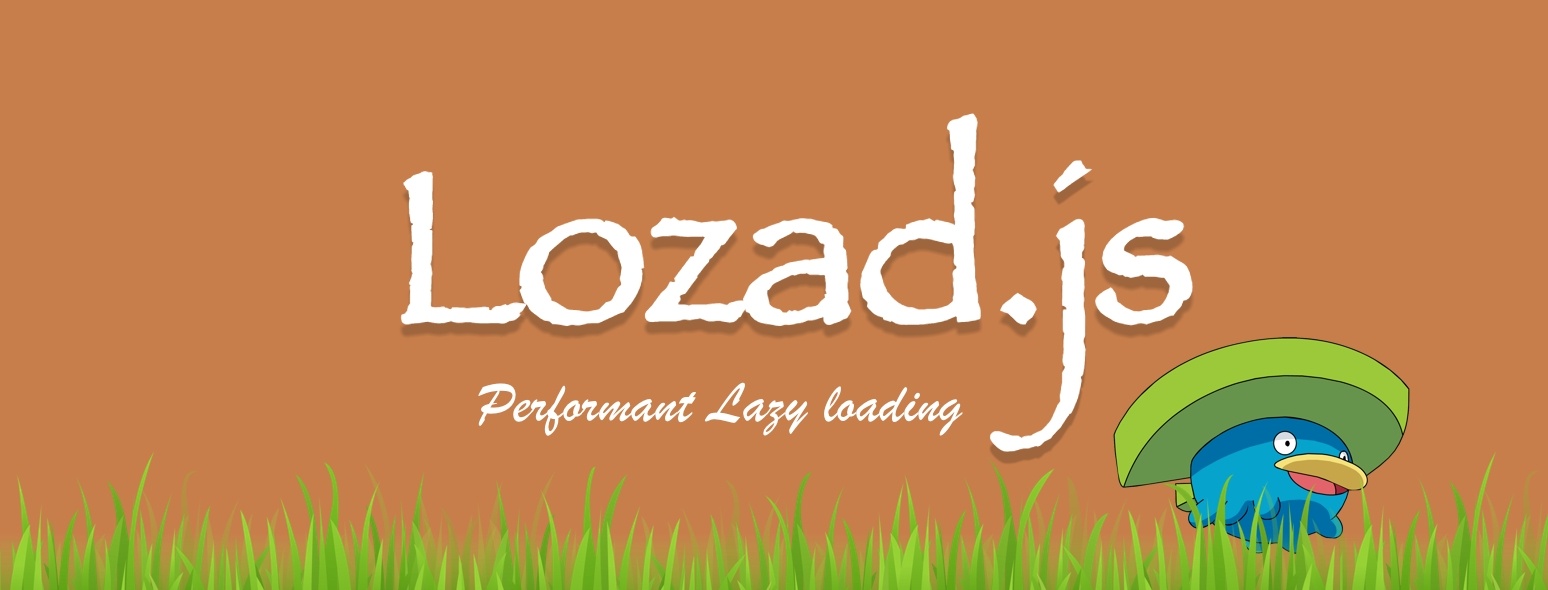
Featured in:
Brands using Lozad.js:
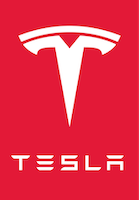
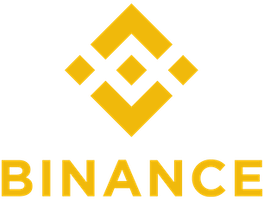
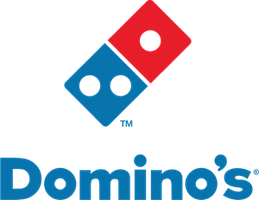
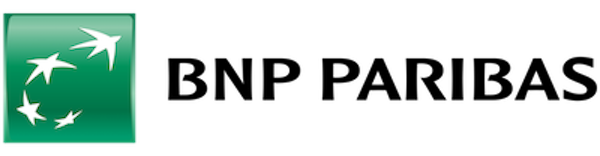
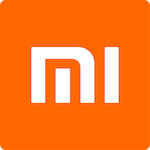
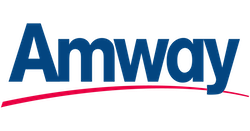
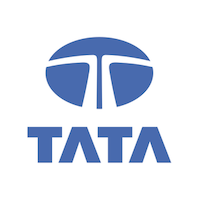
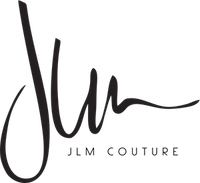
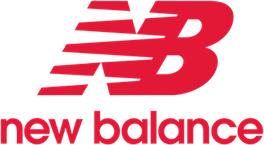
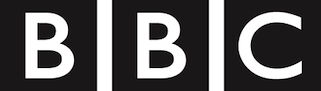
Table of Contents
Yet another Lazy Loading JavaScript library, why?
Install
- ```sh
- # You can install lozad with npm
- $ npm install --save lozad
- # Alternatively you can use Yarn
- $ yarn add lozad
- # Another option is to use Bower
- $ bower install lozad
- ```
- ``` js
- // using ES6 modules
- import lozad from 'lozad'
- // using CommonJS modules
- var lozad = require('lozad')
- ```
- ``` html
- <script type="text/javascript" src="https://cdn.jsdelivr.net/npm/lozad/dist/lozad.min.js"></script>
- ```
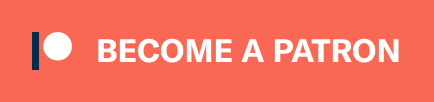
Usage
- ``` html
- <img class="lozad" data-src="image.png">
- ```
- ``` js
- const observer = lozad(); // lazy loads elements with default selector as '.lozad'
- observer.observe();
- ```
- ``` js
- const el = document.querySelector('img');
- const observer = lozad(el); // passing a `NodeList` (e.g. `document.querySelectorAll()`) is also valid
- observer.observe();
- ```
- ``` js
- const observer = lozad('.lozad', {
- rootMargin: '10px 0px', // syntax similar to that of CSS Margin
- threshold: 0.1, // ratio of element convergence
- enableAutoReload: true // it will reload the new image when validating attributes changes
- });
- observer.observe();
- ```
- ``` js
- lozad('.lozad', {
- load: function(el) {
- console.log('loading element');
- // Custom implementation to load an element
- // e.g. el.src = el.getAttribute('data-src');
- }
- });
- ```
Note: The "data-loaded"="true" attribute is used by lozad to determine if an element has been previously loaded.
- ``` js
- lozad('.lozad', {
- loaded: function(el) {
- // Custom implementation on a loaded element
- el.classList.add('loaded');
- }
- });
- ```
- ``` js
- const observer = lozad();
- observer.observe();
- // ... code to dynamically add elements
- observer.observe(); // observes newly added elements as well
- ```
- ``` html
- <img class="lozad" data-src="image.png" data-srcset="image.png 1000w, image-2x.png 2000w">
- ```
- ``` html
- <div class="lozad" data-background-image="image.png">
- </div>
- ```
- ``` html
- <div class="lozad" data-background-image="path/to/first/image,path/to/second/image,path/to/third/image">
- </div>
- ```
- ``` html
- <div class="lozad" data-background-image-set="url('photo.jpg') 1x, url('photo@2x.jpg') 2x">
- </div>
- ```
- ``` html
- <div
- class="lozad"
- data-background-image="/first/custom,image,path/image.png-/second/custom,image,path/image.png"
- data-background-delimiter="-"
- >
- </div>
- ```
- ``` js
- const observer = lozad();
- observer.observe();
- const coolImage = document.querySelector('.image-to-load-first');
- // ... trigger the load of a image before it appears on the viewport
- observer.triggerLoad(coolImage);
- ```
Large image improvment
- ``` html
- <img class="lozad" data-placeholder-background="red" data-src="image.png">
- ```
Example with picture tag
IE browser don't support picture tag!
You need to set data-iesrc attribute (only for your picture tags) with source for IE browser
data-alt attribute can be added to picture tag for use in alt attribute of lazy-loaded images
- ``` html
- <picture class="lozad" style="display: block; min-height: 1rem" data-iesrc="images/thumbs/04.jpg" data-alt="">
- <source srcset="images/thumbs/04.jpg" media="(min-width: 1280px)">
- <source srcset="images/thumbs/05.jpg" media="(min-width: 980px)">
- <source srcset="images/thumbs/06.jpg" media="(min-width: 320px)">
- </picture>
- ```
- ``` html
- <picture class="lozad" style="display: block; min-height: 1rem" data-iesrc="images/thumbs/04.jpg" data-alt="">
- <source srcset="images/thumbs/04.jpg" media="(min-width: 1280px)">
- <source srcset="images/thumbs/05.jpg" media="(min-width: 980px)">
- <source srcset="images/thumbs/06.jpg" media="(min-width: 320px)">
- <img src="data:image/jpeg;base64,/some_lqip_in_base_64==">
- </picture>
- ```
Example with video
- ``` html
- <video class="lozad" data-poster="images/backgrounds/video-poster.jpeg">
- <source data-src="video/mov_bbb.mp4" type="video/mp4">
- <source data-src="video/mov_bbb.ogg" type="video/ogg">
- </video>
- ```
Example with iframe
- ``` html
- <iframe data-src="embed.html" class="lozad"></iframe>
- ```
Example toggling class
- ``` html
- <div data-toggle-class="active" class="lozad">
- </div>
- ```